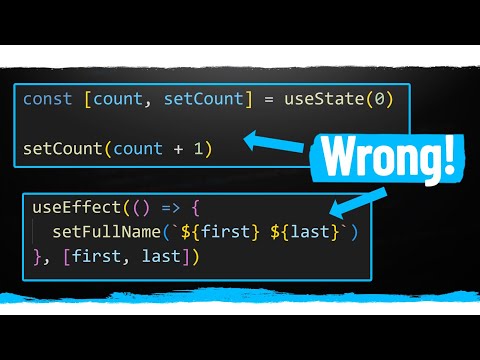
Consider if a state is really needed, or it can be transformed from other states. To prevent unnecessary DOM updates.
If wanna hook a DOM element instead of control it by a state, you can use
useRef()
, likeconst textfieldEmailRef = useRef();
When a state's update relies on its current value, for example:
const [count, setCount] = useState(0);
Don't use
setCount(count + 1);
which may lead to wired behavior like:
// count: 0 setCount(count + 1); setCount(count + 1); console.log(count); // 0
Because the value of
count
is captured when the component is mountedInstead, use:
setCount(current => { return current + 1; }); // It would be fine to do more the same
When using
useEffect
, we might need to set some condition when the useEffect
actually takes effect, like:const [age, setAge] = useAge(0); const [name, setName] = useName(""); const person = { name, age }; useEffect(() => { // do something }, [person]);
However, unfortunately, the condition we specify would not work
Because the condition comparison
useEffect
use is reference comparison, and the variable person
would be a brand new object every time the component is mountedSolution:
Use
useMemo
const person = useMemo(() => { return { name, age }; }, [name, age]);
Use
useEffect
's return value (clean up function), to clean up the context when component is about to unmount, such as the ongoing network requests